If you want to get hands-on experience building great solutions, Azure Accelerator will give you a kickstart. Visit https://www.azureiotsolutions.com and start a great solution in a few seconds.
Try Remote Monitoring
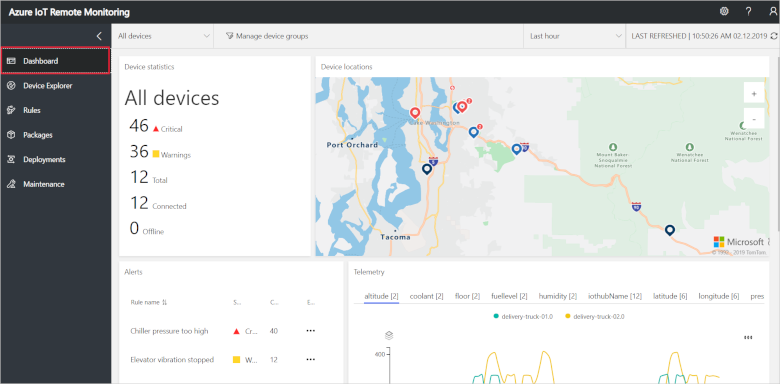
Microsoft Amalga
Microsoft Amalga helps healthcare organisations connect information across different parts of the healthcare system to impact patient outcomes.Visit the website
|
public static bool DisplayFileFromServer(Uri serverUri)
{
// The serverUri parameter should start with the ftp:// scheme.
if (serverUri.Scheme != Uri.UriSchemeFtp)
{
return false;
}
// Geting the object used to communicate with the server.
WebClient WCrequest = new WebClient();
// Credentials
WCrequest.Credentials = new NetworkCredential ("user","password");
try
{
byte [] newFileData = WCrequest.DownloadData (serverUri.ToString());
string fileString = System.Text.Encoding.UTF8.GetString(newFileData);
Console.WriteLine(fileString);
}
catch (WebException e)
{
Console.WriteLine(e.ToString());
}
return true;
}
public static bool DeleteFileOnServer(Uri serverUri)
{
// The serverUri parameter should use the ftp:// scheme.
// It contains the name of the server file that is to be deleted.
// Example: ftp://contoso.com/someFile.txt.
//
if (serverUri.Scheme != Uri.UriSchemeFtp)
{
return false;
}
// Get the object used to communicate with the server.
FtpWebRequest FWRrequest = (FtpWebRequest)WebRequest.Create(serverUri);
FWRrequest.Method = WebRequestMethods.Ftp.DeleteFile;
FtpWebResponse FWRresponse = (FtpWebResponse) FWRrequest.GetResponse();
Console.WriteLine("File delete status: {0}",FWRresponse.StatusDescription);
FWRresponse.Close();
return true;
}